Image AI Analyzer
Check my live project: https://nk-image-ai-analyzer.vercel.app/
Introduction
The Image Analyzer project is an innovative AI-driven application designed to enhance user interaction by leveraging the capabilities of the Gemini API. This tool allows users to upload images, which are then analyzed to extract relevant keywords and generate related content. Upon clicking any of the suggested keywords, the application seamlessly creates detailed textual content tailored to that keyword, offering users in-depth information and insights. Additionally, the project identifies related questions tied to the keywords, fostering a more comprehensive understanding of the subject matter. When users engage with these questions, the app generates content that addresses the inquiries, providing informative and contextually relevant answers. This dual functionality not only enriches the user experience but also facilitates learning and exploration through visual content. The interface is designed to be user-friendly, ensuring that users can easily navigate through their uploaded images and the generated keywords or questions. Built using .tsx files and .env configurations, the application maintains a robust structure that supports scalability and performance. The integration with Firebase allows for efficient data management and real-time updates, making the content generation process dynamic and responsive. Users benefit from a rich database of related topics and questions, enabling a deeper dive into their areas of interest. As they interact with the application, they can expect an engaging and informative experience, whether they are seeking to understand an image, find related concepts, or generate content for educational or creative purposes. This project exemplifies the potential of AI in bridging visual media with textual information, ultimately enhancing learning and engagement across various domains. With continuous updates and improvements planned for the future, the Image Analyzer aims to become a leading tool in AI-driven content generation and image analysis, catering to a diverse audience from students to professionals seeking to enrich their knowledge base.
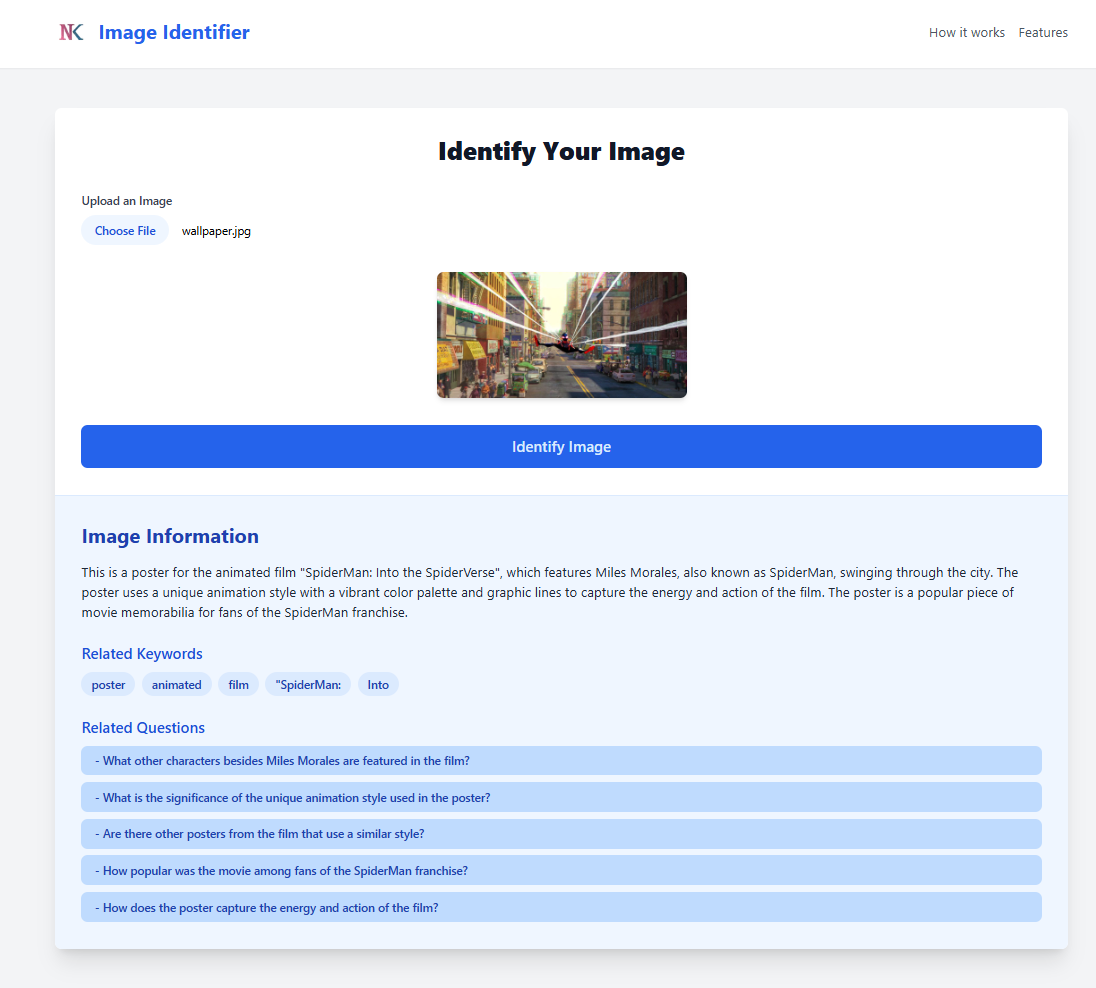
Install packages
To make this project successful, you need to install some libraries and packages.Open the terminal and install all the necessary libraries listed below.
Next JS App
The command npx create-next-app@latest is used to quickly scaffold a new Next.js application, which is a popular React framework for building server-rendered and statically generated web applications. By utilizing npx, which allows you to execute packages from the npm registry without globally installing them, this command fetches the latest version of the create-next-app package.
npx create-next-app@latest
Google AI
The command npm install @google/generative-ai installs the Google Generative AI package, which provides tools and APIs for building applications that leverage generative AI models. This package facilitates the integration of advanced AI capabilities, enabling developers to create features like text generation, image synthesis, and more within their applications.
npm install @google/generative-ai
React Icons
The command npm install react-icons --save is used to install the react-icons library in a React project. This library provides a collection of popular icons from various icon sets, allowing developers to easily integrate scalable vector icons into their applications. By using the --save flag (now default in newer versions of npm), the library is added as a dependency in the package.json file, ensuring that it is included in the project's build process and can be easily managed. After installation, you can import specific icons from the library and use them as React components in your project.
npm install react-icons --save
Create Gemini API Key
- Go to and SignIn in Google AI for developers website https://ai.google.dev/
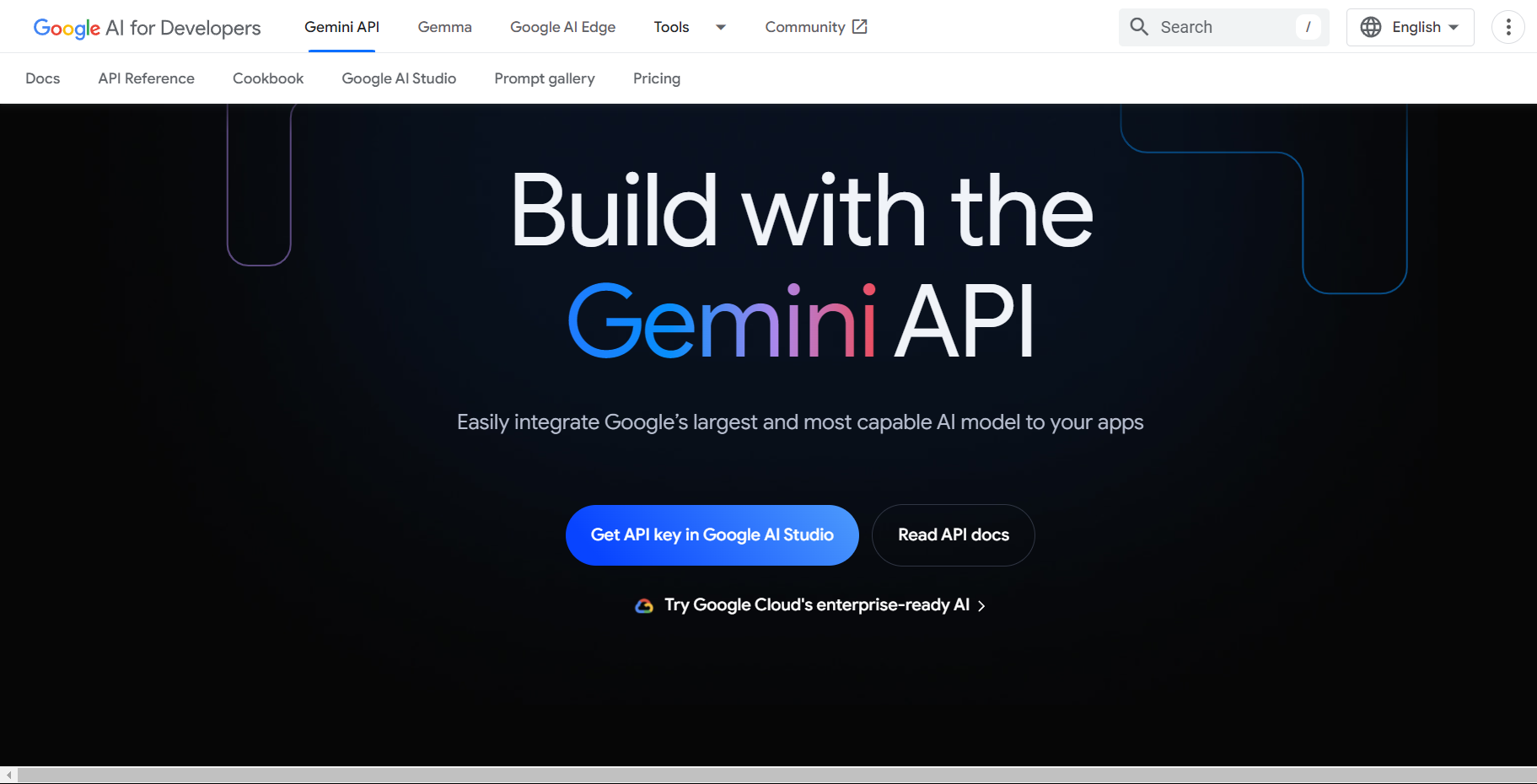
- Click Get API Key In Google AI Studio.
- Click The Create API Key and give the project name.
- Now Your API Key is created Successfully. Copy the API Key and replace it in the API_KEY variable.
Header
This header component, designed for the Image AI Analyzer project, offers a clean and responsive navigation experience. It features a sleek logo and title combination, emphasizing a professional and modern look with a minimalist blue and white color scheme. The navigation menu includes key sections like "Home," "How it works," and "Features," allowing users to easily access core areas of the application. Built using React and Next.js with Tailwind CSS for styling, this header ensures smooth transitions and hover effects, enhancing user interaction. Its responsive design ensures optimal viewing across different devices and screen sizes.
"use client" import Link from 'next/link' import React from 'react' import Image from 'next/image' export const Header = () => { return ( <header className="bg-white shadow-sm"> <div className="max-w-7xl mx-auto px-4 sm:px-6 lg:px-8 py-6"> <div className="flex items-center justify-between"> {/* logo */} <div className="flex items-center"> <Image src={"/file.png"} alt="image" width={40} height={40} className="mr-3" /> <h1 className="text-2xl font-bold text-blue-600"> Image Identifier </h1> </div> {/* menus */} <nav> <ul className="flex space-x-4"> <Link href={"#"} className="text-gray-600 hover:text-blue-600 transition duration-150 ease-in-out"> Home</Link> <Link href={"#how-it-works"} className="text-gray-600 hover:text-blue-600 transition duration-150 ease-in-out"> How it works</Link> <Link href={"#features"} className="text-gray-600 hover:text-blue-600 transition duration-150 ease-in-out"> Features</Link> </ul> </nav> </div> </div> </header> ) } export default Header;
Main-Container
The Image AI Analyzer project utilizes advanced AI technology to identify and analyze images, providing detailed information and insights about their content. This tool allows users to upload an image, and through the power of Google's Generative AI (Gemini model), it extracts key data, generates keywords, and even suggests related questions based on the image. The system is designed for fast, accurate results with a user-friendly interface, making it easy to navigate and explore various image details.
"use client"; import React, { useState } from 'react'; import Image from 'next/image'; import { GoogleGenerativeAI } from '@google/generative-ai'; export const MainContainer = () => { const [image, setImage] = useState<File | null>(null); const [loading, setLoading] = useState(false); const [result, setResult] = useState<string | null>(null); const [keywords, setKeywords] = useState<string[]>([]); const [relatedQuestion, setRelatedQuestion] = useState<string[]>([]); const identifyImage = async (additionalPrompt: string = "") => { if (!image) return; setLoading(true); const genAI = new GoogleGenerativeAI( process.env.NEXT_PUBLIC_GOOGLE_API_KEY! ); const model = genAI.getGenerativeModel({ model: "gemini-1.5-flash", }); try { const imageParts = await fileToGenerativePart(image); const result = await model.generateContent([ `Identify this image and provide its name and important information, including a brief explanation about that image. ${additionalPrompt}`, imageParts, ]); const response = await result.response; const text = response .text() .trim() .replace(/'''/g, "") .replace(/\*\*/g, "") .replace(/\*/g, "") .replace(/-\s*/g, "") .replace(/\n\s*\n/g, "\n"); setResult(text); generateKeywords(text); await generateRelatedQuestions(text); } catch (error) { console.log((error as Error)?.message); } finally { setLoading(false); } }; const fileToGenerativePart = async (file: File): Promise<{ inlineData: { data: string; mimeType: string }; }> => { return new Promise((resolve, reject) => { const reader = new FileReader(); reader.onloadend = () => { const base64Data = reader.result as string; const base64Content = base64Data.split(", ")[1]; resolve({ inlineData: { data: base64Content, mimeType: file.type, }, }); }; reader.onerror = reject; reader.readAsDataURL(file); }); }; const handleImageUpload = (e: React.ChangeEvent<HTMLInputElement>) => { if (e.target.files && e.target.files[0]) { setImage(e.target.files[0]); } }; const generateKeywords = (text: string) => { const words = text.split(/\s+/); const keywordsSet = new Set<string>(); words.forEach(word => { if (words.length > 4 && ![ "this", "that", "with", "from", "have", "is", "a", "are", "was", "the", "of", "what", "which", "for", "at", "an" ].includes(word.toLowerCase())) { keywordsSet.add(word); } }); setKeywords(Array.from(keywordsSet).slice(0, 5)); }; const regenerateContent = (Keyword: string) => { identifyImage(`Focus more on aspects related to this "${Keyword}".`,); }; const generateRelatedQuestions = async (text: string) => { const genAI = new GoogleGenerativeAI( process.env.NEXT_PUBLIC_GOOGLE_API_KEY! ); const model = genAI.getGenerativeModel({ model: "gemini-1.5-flash", }); try { const result = await model.generateContent([ `Based on the following information about an image, generate 5 related questions that someone might ask to learn more about the subject: ${text} Format the output as a simple list of questions, one per line`, ]); const response = await result.response; const questions = response.text().trim().split("\n"); setRelatedQuestion(questions); } catch (error) { console.log((error as Error)?.message); setRelatedQuestion([]); } }; const askRelatedQuestion = (question: string) => { identifyImage(`Answer the following question about the image: "${question}".`,); } return ( <main className="max-w-7xl mx-auto px-4 sm:px-6 lg:px-8 py-12"> <div className='bg-white rounded-lg shadow-xl overflow-hidden'> <div className='p-8'> <h2 className='text-3xl font-extrabold text-gray-900 mb-8 text-center'> Identify Your Image </h2> <div className='mb-8'> <label htmlFor='image-upload' className='block text-sm font-medium text-gray-700 mb-2'> Upload an Image </label> <input type='file' id="image-upload" accept="image/*" onChange={handleImageUpload} className='block w-full text-sm to-gray-500 file:mr-4 file:py-2 file:px-4 file:rounded-full file:border-0 file:text-sm file:font-semibold file:bg-blue-50 file:text-blue-700 hover:file:bg-blue-100 transition duration-150 ease-in-out file:cursor-pointer' /> </div> {/* display the image */} {image && ( <div className='mb-8 flex justify-center'> <Image src={URL.createObjectURL(image)} alt="uploaded img" width={300} height={300} className='rounded-lg shadow-md' /> </div> )} <button type='button' onClick={() => identifyImage()} disabled={!image || loading} className='w-full bg-blue-600 text-blue-100 py-3 px-4 rounded-lg hover:bg-blue-700 transition duration-150 ease-in-out disabled:opacity-50 disabled:cursor-not-allowed font-medium text-lg'> {loading ? "Identifying..." : "Identify Image"} </button> </div> {result && ( <div className='bg-blue-50 p-8 border-t border-blue-100'> <h3 className='text-2xl font-bold text-blue-800 mb-4'>Image Information</h3> <div className='max-w-none'> {result.split("\n").map((line, index) => { if (line.startsWith("Important Information:") || line.startsWith("Other Information:")) { return ( <h4 className='text-xl font-semibold mt-4 mb-2 text-blue-700' key={index}>{line}</h4> ) } else if (line.match(/^\d+\./) || line.startsWith("-")) { return ( <li key={index} className='ml-4 mb-2 text-gray-700'>{line}</li> ); } else if (line.trim() !== "") { return ( <p key={index} className='mb-2 text-gray-800'>{line}</p> ); } return null; })} </div> <div className='mt-6'> <h4 className='text-lg font-semibold mb-2 text-blue-700'>Related Keywords</h4> <div className='flex flex-wrap gap-2'> {keywords.map((keyword, index) => ( <button type='button' key={index} onClick={() => regenerateContent(keyword)} className='bg-blue-100 text-blue-800 px-3 py-1 rounded-full text-sm font-medium hover:bg-blue-200 trasnsition duration-150 ease-in-out'>{keyword}</button> ))} </div> </div> {relatedQuestion.length > 0 && ( <div className='mt-6'> <h4 className='text-lg font-semibold mb-2 text-blue-700'>Related Questions</h4> <ul className='space-y-2'> {relatedQuestion.map((question, index) => ( <button type='button' onClick={() => askRelatedQuestion(question)} className='text-left w-full bg-blue-200 text-blue-800 px-4 py-2 rounded-lg text-sm font-medium hover:bg-blue-300 transition duration-150 ease-in-out'>{question}</button> ))} </ul> </div> )} </div> )} </div> <section id="how-it-works" className='mt-16'> <h2 className='text-3xl font-extrabold text-gray-900 mb-8 text-center'> How It Works </h2> <div className='grid grid-cols-1 md:grid-cols-3 gap-8'> {[{ title: "Upload Image", description: "Start by uploading an image in any of the supported formats. Make sure the file size is within the limits." }, { title: "AI Analysis", description: "Our advanced AI will analyze the uploaded image and extract detailed information about its contents." }, { title: "Get Results", description: "Once the analysis is complete, you will receive the results with a breakdown of the image's contents." }].map((step, index) => ( <div key={index} className='bg-white rounded-lg shadow-md p-6 transition duration-150 ease-in-out hover:scale-105 cursor-pointer'> <div className='text-3xl font-semibold text-blue-600 mb-4'> {index + 1} </div> <h3 className='text-xl font-semibold mb-2 text-gray-800'>{step.title}</h3> <p className='text-gray-600'>{step.description}</p> </div> ))} </div> </section> <section id="features" className='mt-16'> <h2 className='text-3xl font-extrabold text-gray-900 mb-8 text-center'> Features </h2> <div className='grid grid-cols-1 md:grid-cols-2 gap-8'> {[{ title: "Accurate Identification", description: "Our AI system provides precise and reliable image identification, ensuring you get accurate results every time." }, { title: "Detailed Information", description: "Receive in-depth analysis with detailed information about every aspect of your uploaded image." }, { title: "Fast Results", description: "Enjoy quick processing and get your image analysis results in a matter of seconds, saving you time." }, { title: "User Friendly Interface", description: "Navigate through our intuitive and user-friendly interface with ease, making the experience seamless." }].map((feature, index) => ( <div key={index} className='bg-white rounded-lg shadow-md p-6 transition duration-150 ease-in-out hover:scale-105 cursor-pointer'> <h3 className='text-xl font-semibold mb-2 text-blue-600'>{feature.title}</h3> <p className='text-gray-600'>{feature.description}</p> </div> ))} </div> </section> </main> ); };
Footer
This footer component, designed for the Image AI Analyzer project, offers a clean and responsive navigation experience. It features a structured layout with sections like "About Us," "Quick Links," "Our Projects," and "Follow Us." Each section is clearly labeled and provides essential information or links, enhancing user accessibility. Built using React and Next.js with Tailwind CSS for styling, this footer ensures smooth transitions and hover effects for interactive elements. Its responsive design guarantees optimal viewing across various devices and screen sizes.
"use client"; import Link from 'next/link' import { FaWhatsapp, FaLinkedin, FaGithub, FaTelegram } from 'react-icons/fa'; import { IoMdMailOpen } from 'react-icons/io' ; export const Footer = () => { return ( <footer className="bg-white shadow-sm mt-16"> <div className="max-w-7xl mx-auto px-4 sm:px-6 lg:px-8 py-8"> <div className="grid grid-cols-1 md:grid-cols-4 gap-8"> {/* About Section */} <div> <h3 className="text-lg font-semibold text-blue-600 mb-4">About Us</h3> <p className="text-gray-600"> Image Identifier uses advanced AI technology to provide accurate image analysis, giving detailed results in seconds. </p> </div> {/* Quick Links Section */} <div> <h3 className="text-lg font-semibold text-blue-600 mb-4">Quick Links</h3> <ul className="text-gray-600 space-y-2"> <li> <Link href={"#"} className="hover:text-blue-600 transition duration-150 ease-in-out"> Home </Link> </li> <li> <Link href={"#how-it-works"} className="hover:text-blue-600 transition duration-150 ease-in-out"> How it Works </Link> </li> <li> <Link href={"#features"} className="hover:text-blue-600 transition duration-150 ease-in-out"> Features </Link> </li> </ul> </div> {/* Our Projects Section */} <div> <h3 className="text-lg font-semibold text-blue-600 mb-4">Our Projects</h3> <ul className="text-gray-600 space-y-2"> <li> <a href="https://nk-codepen-clone.web.app/" target='blank' className="hover:text-blue-600 transition duration-150 ease-in-out"> Online Coding Platform </a> </li> <li> <a href="https://nirmalkumarofllll.github.io/Portfolio/IDS.html" target='blank' className="hover:text-blue-600 transition duration-150 ease-in-out"> IDS </a> </li> <li> <a href="https://nirmalkumarofllll.github.io/Portfolio/CRS.html" target='blank' className="hover:text-blue-600 transition duration-150 ease-in-out"> CRS </a> </li> <li> <a href="https://nirmalkumarofllll.github.io/Portfolio/Chatbot.html" target='blank' className="hover:text-blue-600 transition duration-150 ease-in-out"> Chatbot </a> </li> </ul> </div> {/* Social Media Section */} <div> <h3 className="text-lg font-semibold text-blue-600 mb-4">Follow Us</h3> <div className="flex space-x-4"> <a href="https://mail.google.com/mail/u/0/?fs=1&to=nirmalkumarofllll@gmail.com&tf=cm" target='blank' className="hover:text-blue-600 transition duration-150 ease-in-out"> <IoMdMailOpen size={24} /> </a> <a href="https://www.linkedin.com/in/nirmalkumarp-ofllll/" target='blank' className="hover:text-blue-600 transition duration-150 ease-in-out"> <FaLinkedin size={24} /> </a> <a href="https://github.com/Nirmalkumarofllll" target='blank' className="hover:text-blue-600 transition duration-150 ease-in-out"> <FaGithub size={24} /> </a> <a href="https://wa.me/8220694842" target='blank' className="hover:text-blue-600 transition duration-150 ease-in-out"> <FaWhatsapp size={24} /> </a> <a href="https://t.me/Nirmal_offl" target='blank' className="hover:text-blue-600 transition duration-150 ease-in-out"> <FaTelegram size={24} /> </a> </div> </div> </div> <div className="mt-8 border-t pt-6 text-center text-gray-500 text-sm"> © {new Date().getFullYear()} Nirmal Kumar. All rights reserved. </div> </div> </footer> ); }; export default Footer;
Download Project
You can download the complete project files here. This Image AI Analyzer project is connected with the Gemini API to enhance user experience with AI-driven image analysis. The project allows users to upload images, and in return, it generates relevant keywords and related questions based on the analysis of the image. When users click on these generated keywords or questions, the system dynamically creates detailed content associated with them. This intuitive AI integration helps users gain insights and information quickly and efficiently, making it an excellent tool for content creators, researchers, and developers.
Download ProjectNOTE: Replace with your Gemini API key before run the code. Hide your API key before posting it in the Github or in any other platforms.